Overview
Recruit Me is a desktop application designed for Recruitment Companies looking to store their client’s data ergonomically. It is an adaptation of AddressBook - Level 4 which is a desktop address book application used for teaching Software Engineering principles. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: added the ability to sort persons through various means
-
Sorts can be made for all fields useful to a recruiting agency employee (
name
;surname
;education
;gpa
;degree
;skills
;positions
;endorsements
;skill number
;position number
;endorsement number
) and the ability to sort them in reverse order. -
What it does: allows the user to sort the list of persons.
-
Justification: This feature allows the user to sort through the client’s data. It is pivotal in transforming the AddressBook to a Recruiting platform since it provides the user with multiple tools for visually seeing their clients in a logical manner.
-
Highlights: This enhancement is fully compatible with the other commands and proves particularly useful to the user when used in conjunction with the
filter
command. It also employs intelligent sorting meaning that one sorting method can utilise another sorting method to further sort similar persons.
-
-
Minor enhancement:
-
changes to VersionedAddressBook and ModelManager to allow for deleting all persons at once and adding a person with the filter in place.
-
multiple changes to tests and style to aid the building of Travis.
-
Contributions to the User Guide
-
Addition of sort method description in a new section (Recruit Me - User Guide/Features/Sorting Persons: sort).
-
The full utility is explained to ensure the user can get a grasp of the new tools available to them.
Contributions to the Developer Guide
-
The steps required for the addition of a new sort method is outlined for future developers in a new section (Recruit Me - Developer Guide/Implementation/Sort Commands).
-
A full description of the workings of the sort command has been provided.
-
Steps for including a new sort command has been outlined.
-
Some rational for the sort command has been outlined.
Sort Commands
Current Implementation Summary
There are currently eleven main sorting methods present: name
, surname
, gpa
, education
, degree
, skills
, positions
, endorsements
, skill number
, position number
, endorsement number
.
There is also a complimentary reverse
sort method for each main sorting method.
-
name is called by the user through the following cli input:
sort name
.
It takes the current list displayed in the left hand GUI panel and sorts them by name alphabetically. The name sort begins with the first name and then proceeds to last name. -
surname is called by the user through the following cli input:
sort surname
.
It takes the current list displayed in the left hand GUI panel and sorts them by surname alphabetically. -
gpa is called by the user through the following cli input:
sort gpa
.
It takes the current list displayed in the left hand GUI panel and sorts them by gpa in decreasing numeric order. -
education is called by the user through the following cli input:
sort education
.
It takes the current list displayed in the left hand GUI panel and sorts them by education alphabetically. -
degree is called by the user through the following cli input:
sort degree
.
It takes the current list displayed in the left hand GUI panel and sorts them by degree starting with PhD and then proceeding to Masters, Bachelors, Associates and High School in this order. -
skills is called by the user through the following cli input:
sort skills
.
It takes the current list displayed in the left hand GUI panel and first orders the skill tags for each person alphabetically. The method then proceeds to sort all persons based on their skill tags, in alphabetical order. -
positions is called by the user through the following cli input:
sort positions
.
It takes the current list displayed in the left hand GUI panel and first orders the position tags for each person alphabetically. The method then proceeds to sort all persons based on their position tags, in alphabetical order. -
endorsements is called by the user through the following cli input:
sort endorsements
.
It takes the current list displayed in the left hand GUI panel and first orders the endorsements for each person alphabetically. The method then proceeds to sort all persons based on their endorsements, in alphabetical order. -
skill number is called by the user through the following cli input:
sort skill number
.
It takes the current list displayed in the left hand GUI panel and orders the persons based on their number of skills in descending order. -
position number is called by the user through the following cli input:
sort position number
.
It takes the current list displayed in the left hand GUI panel and orders the persons based on their number of positions in descending order. -
endorsement number is called by the user through the following cli input:
sort endorsement number
.
It takes the current list displayed in the left hand GUI panel and orders the persons based on their number of endorsements in descending order. -
reverse can be applied before the sort keyword (e.g.
name
) through the following cli input:sort reverse name
(the current list means that if filter is on, only those filtered persons shall be sorted and the filter shall remain on)
Intelligent Sorting:
For a recruiting company with a large number of contacts it is highly likely that certain fields shall be repeated (for example two different persons can both have Bachelors degrees). To aid the user, intelligent sorting has been implemented. It sorts persons that match in the field that the initial sort is looking at and then performs a secondary sort using a method related to the initial sort. The sequences of these are shown below.
Initial Sort Method |
Subsequent Sort Method (for persons with same value after first sort method) |
Secondary Subsequent Sort Method (for persons with same value after first and subsequent sort method) |
Name |
- |
- |
Surname |
Name |
- |
Skills, Positions, Endorsements |
Name |
- |
Skills, Positions, Endorsements Number |
Skills, Positions, Endorsements respectively |
Name |
Gpa |
Name |
- |
Degree |
GPA |
Name |
Education |
GPA |
Name |
Current Implementation Description.
The sort command works in a similar fashion to the commands already in place. Below is a sequence diagram for a general sort method.
The SortCommandParser
implements the already created Parser
interface. It is used to take the CLI user input and make a new SortCommand object only if a valid Sort Word has been passed into the CLI.
The SortCommand is an extension of the abstract command
class. This means history, command results and addressbook committing follow the same format as the commands in place. This allows it’s compatibility with those commands already present.
It takes the list of persons in the addressbook. It ensures that if a filter
is present, only these filtered persons are sorted, but the complete persons list is left unaffected. This presents accidental deletion of contact information.
The SortCommand class proceeds to take the input, initially from the CLI, and uses processSortMethod
to call the necessary sorting method.
The sorting method classes all implement the SortMethod interface which executes the necessary sort on the list and has a getList() method.
Once a correctly ordered list of persons has been retrieved by the SortCommand class, it checks for the reverse
keyword and makes the necessary adaption.
All the persons are then deleted from this model. Due to the severity of this command, additional care has been taken to ensure it can only be processed providing there is a list of persons (of the same length) present to replace it.
It then adds the persons back into the addressbook in the correct order, taking note of the filter to ensure compatibility.
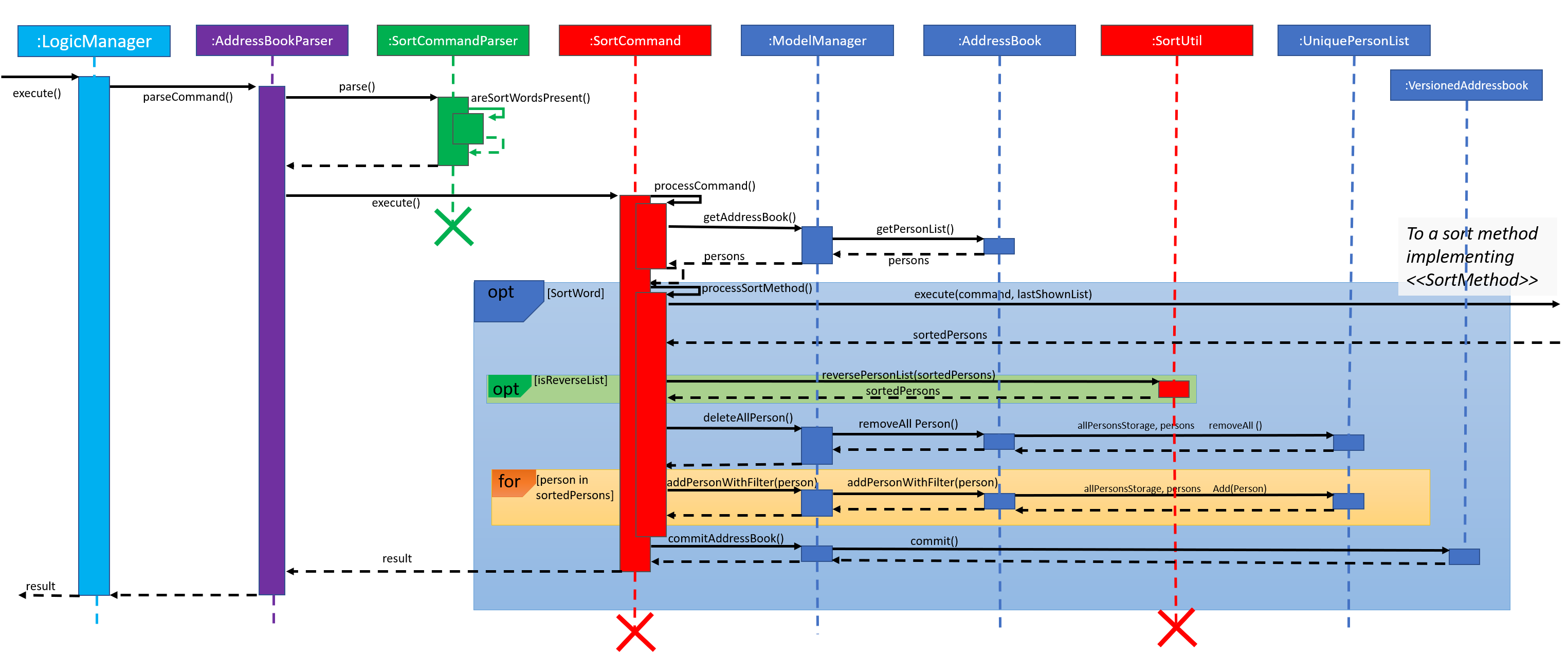
Also of note are the methods used in the sorts themselves. Below is shown the sequence diagram for a surname sorting method as an example.
The sort methods begin by retrieving the field of interest to the particular sorting method. The aim is to create a Comparator<Person>
which can subsequently be easily sorted either numerically or alphabetically.
To retrieve the field of interest, the Person
class is used. For those fields not suited to numerical or alphabetical sorting, associated values can be generated. For example in the degree
field, values are given to the different levels which can then be sorted.
These methods should be stored in PersonUtil
to keep the frequently used Person
class as clear and relevant as possible.
For the skills
, positions
and endorsements
sorting methods, it is required for the person’s individual tags to be sorted first. This uses a sorting method on each person and the creation of new person with correctly ordered skill/position/endorsement tags.
Once a comparator has been created, the SortUtil
class can be used to perform the actual sorting. This makes use of Java’s stream.Collectors
method.
For intelligent sorting the SortListWithDuplicates
class is used. It divides the list of persons from the initial sort into smaller lists (dupPersonList) of those returning the same comparator value.
These are then individually sorted by the subsequent sorting method. By utilising similar methods for each sort method, this makes the subsequent calling of further sort methods quick and easy.
It is worth noting that a subsequent sorting method shall then perform a further sort if its comparator returns a duplicate value within the duplicate list.
This process is terminated by the sort name method since the addressbook does not allow people to be added with the same name.
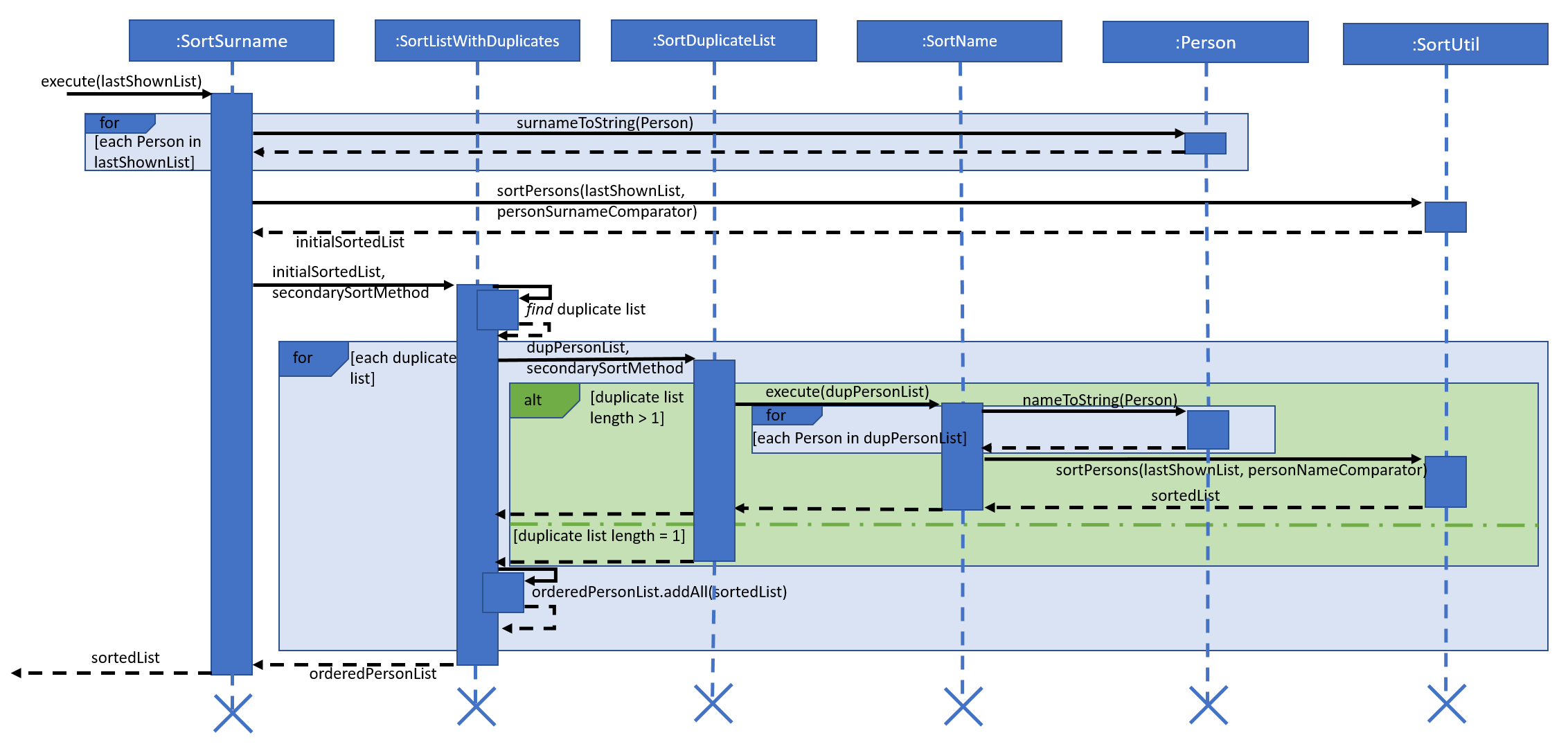
Implementation Rational
Despite the risk of slightly increasing the coupling, the aim was to use methods already written and rely on good cohesion.
For example, once a sort command has correctly written the correctly ordered persons to a List<Person>
, rather than duplicating large amounts of code by modifying the already listed persons in the GUI, it shall simply remove the persons in that addressbook version and then immediately re-add them in the correct order.
It is of note that the temporary deletion of persons from the addressbook should be foolproof and there should be no way that the sort command ever permanently deletes the addressbook. Furthermore, ensuring the command works with the already implemented undo/redo command should ensure the user still has full control over all the persons in the contact book.
The future of this product demands the dynamic addition of contact fields as they become important. These fields should then be able to be sorted. Consequently it is paramount that the addition of a further sort command is easy.
This is achieved through the SortMethod
interface and provision of very adaptable sorting method techniques that can be later used. The steps taken to add a new sort command are provided below.
Approaches Considered
When writing sort commands, there were two approaches considered: modify the indices of all persons and then refresh the left-hand GUI panel with this new list; or temporarily delete the list of persons and then add a new list of correctly ordered persons.
Elements of lists in Java are ordered by when they were added. Sorting is possible using Collections, however this requires them to be strings. Since the Recruit Me application contains lists of various object types, typically Person, there is no immediate compatibility with Collections. Furthermore it was suspected that to simply modify the indices of persons, a lot of duplicate code would be need to be written since this aspect of addressbook-level4 was not easily modifiable. Because of this the second method was opted for.
It was also decided to make this command as similar as possible in style to those already present. This meant that it was almost immediately compatible with the other commands. This way it could also make use of various methods already in place.
Adding a New Sort Command
To add a new sort command, the following classes should be altered accordingly:
-
cliSyntax
- add the necessary new SortWord (and the reverse option), this should match the desired CLI user input -
SortCommandParser
- add the new SortWords as an accepted input -
Sort[NewMethod]
- a new class that implements theSortMethod
interface, that will execute a sort on the list of Persons, according to the new method, and a getList() method for returning this correctly ordered list. Here a subsequent ordering method can be specified for intelligent sorting -
Person
- it is often useful to have a Person’s field as a string or numerical value in order to create a comparator. Consequently a method should be provided here for returning the Person’s new field as a string or number. -
SortCommand
- create the link between the new SortWord and the newSort[NewMethod]
class -
SortUtil
- a place for lower-level processes required by the new sort method and called fromSort[NewMethod]
When choosing the subsequent sort for intelligent sorting, a loop must not be created. For example matching gpa’s can’t use education as a subsequent sorting method if education is already using gpa. This is because if a person has both the same gpa and education, they can’t be sorted.
The developer should also add the necessary testing methods in SortCommandTest
and SortCommandIntegrationTest